How We Built Software for the 2024 MATE ROV Competition
The 2024 MATE ROV competition was an ambitious undertaking for our team. As the software chief, I had the responsibility not only to develop the control system for our ROV, ROVIN, but also to coordinate with our mechanical and electrical departments. Our goal was to create a control system that could manage six degrees of freedom (6DOF), ensuring precise, stable movement while accommodating any changes in hardware or design. Here’s how our team navigated the challenges and brought our vision to life.
Leading a Cross-Functional Team
The software was just one piece of a much larger puzzle. My job went beyond coding—it involved managing the software team and collaborating closely with other departments. For example, when the mechanical team made a change to the thruster configuration, or the electrical team switched out a microcontroller, we had to adapt the software accordingly without compromising the integrity of the system.
This constant communication was essential. We had to ensure that every hardware adjustment—whether it was as significant as changing the microcontroller or as simple as moving a motor—wouldn’t disrupt the control algorithms, especially the equations of motion or the way the microcontrollers interfaced with the system.
Tackling the Challenge Head-On
Building the software was a complex task, but managing a team to do so within the shifting parameters of a cross-functional project added another layer of difficulty. Early on, we realized that modularity and flexibility were essential, not just in the software but across the entire ROV system. This meant working closely with the other departments to ensure the software could adapt to any change they made.
Designing the Software Architecture with Flexibility in Mind
Given the complexity of our ROV system, we knew we had to build a software architecture that could absorb changes from the mechanical and electrical teams without requiring complete rewrites. This involved designing the control system in three layers:
- Surface Components: The driver station communicates with the ROV via a TCP/IP connection. To solve this we created a react app to interface with the ROV.
- Onboard Computer (Jetson Nano): This acts as the processing center for sensor inputs and user commands. One key challenge was ensuring that, even if hardware changes were made, the onboard computer could still translate commands into precise thruster movements.
- Base Controller: This calculates the equations of motion and ensures proper thruster control based on the latest configuration. Whether the mechanical team moved a motor or the electrical team switched a sensor, the Base Controller had to adapt without affecting overall control.
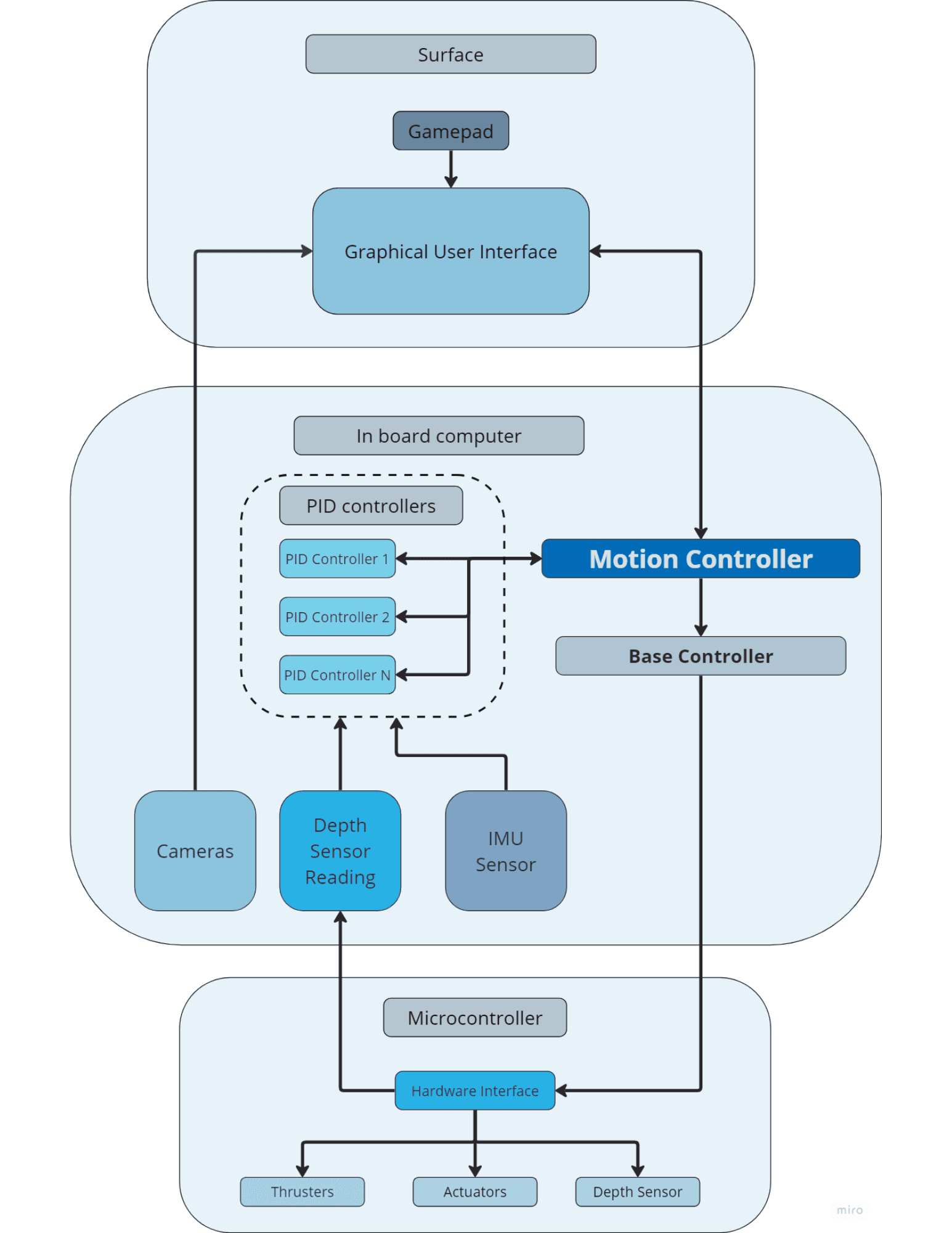
The Base Controller was the most challenging part of the software to develop. It was responsible for calculating the exact thruster outputs needed to achieve the desired movement. This was no small feat given that the ROV needed to be able to move in six degrees of freedom, and the mechanical and electrical teams were constantly making changes to the hardware. The Base Controller had to be able to adapt to these changes and continue to function properly.
Our software works in levels of abstraction to maintain flexibility. At each level, we aimed to abstract away both the hardware and lower-level software details. For instance, the Base Controller is only concerned with controlling the overall movement of the ROV, not how individual motors function.
This abstraction means that when the Base Controller needs to set the velocity for a specific thruster, it doesn’t need to know whether it's communicating with an ESC (Electronic Speed Controller) or a specific microcontroller. We designed it so that you can easily call a function like:
1//...
2//some code of how we control individual thrusters
3thruster_pub_ = nh_.advertise<std_msgs::Float64>("/bottom_front_left_thruster", 10);
4std_msgs::Float64 msg;
5msg.data = target_velocity;
6thruster_pub_.publish(msg);
7//continue
Similarly, we made it such that we could control the overall movement of the ROV (base controller) by calling a function like:
1//...
2//some code of how we control the overall movement of the ROV
3rov_velocity_pub_ = nh_.advertise<geometry_msgs::Twist>("/base", 10);
4geometry_msgs::Twist velocity_msg;
5// Set linear velocities
6velocity_msg.linear.x = some_value;
7velocity_msg.linear.y = some_value;
8velocity_msg.linear.z = some_value;
9
10// Set angular velocities (roll, pitch, yaw)
11velocity_msg.angular.x = some_value;
12velocity_msg.angular.y = some_value;
13velocity_msg.angular.z = some_value;
14
15rov_velocity_pub_.publish(velocity_msg);
Stability and Precision: Sensor Integration and PID Controllers
Stability was critical for our ROV, and it was my job to ensure that the system could remain stable even when other departments made hardware changes. This was achieved through cascading PID controllers (for each degree of freedom), which provided real-time control over ROVIN’s movement.
Driver Station GUI: Creating a User-Friendly Interface
Finally, we knew that the user experience was critical. Working with the mechanical and electrical teams, we built a driver station that could handle the complex control system with ease. The React and Tauri-based GUI was designed to be lightweight and cross-platform, with features that supported intuitive control.
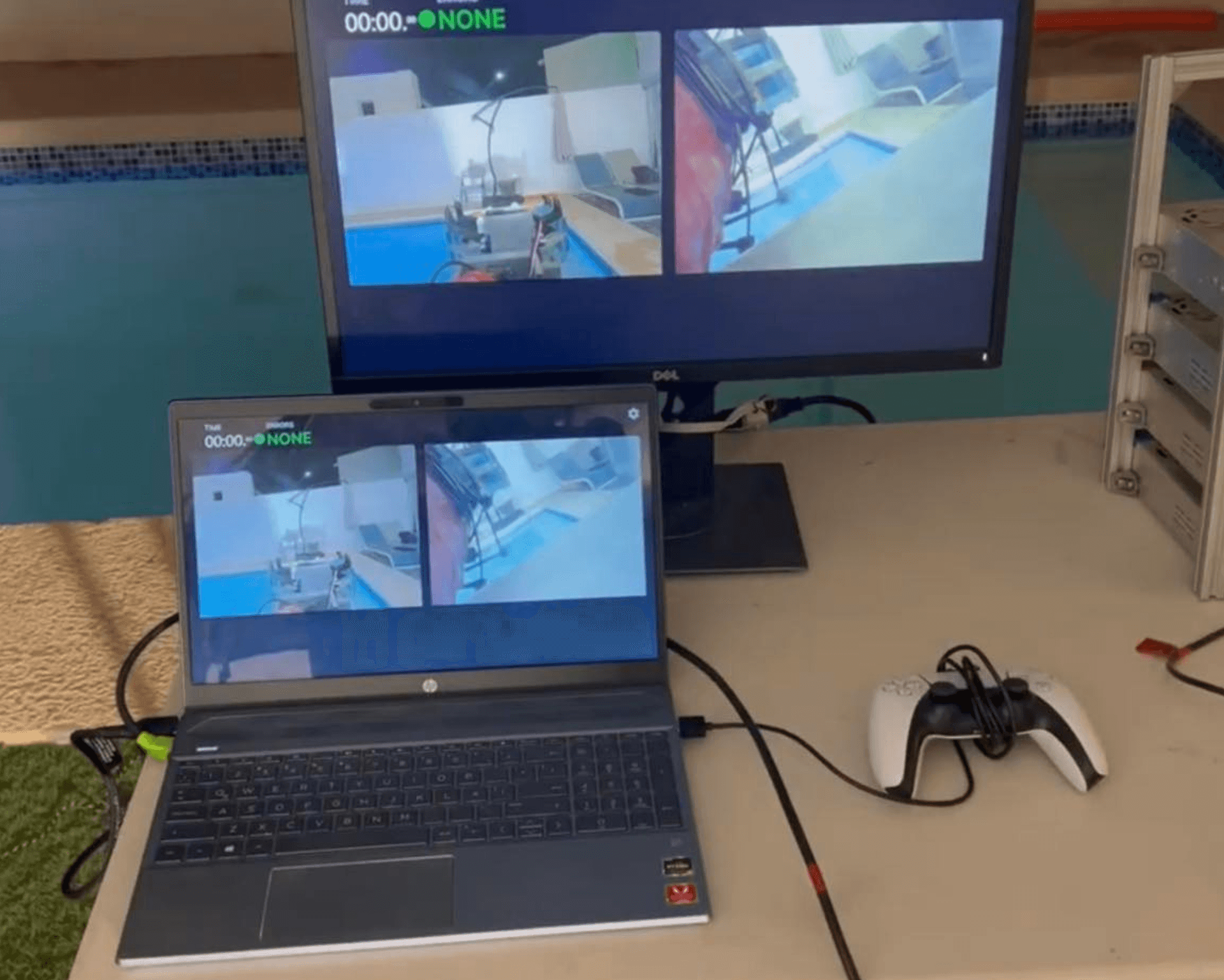
- ROSBridge Integration: We made sure ROSBridge allowed easy communication between the driver station and the ROV, regardless of changes made by the hardware teams.
- Gamepad Support: We integrated gamepad support to make controlling ROVIN as user - friendly as possible, even for team members with minimal training.
Leading the Team to Success: Lessons Learned
Managing the software for the 2024 MATE ROV competition was about more than just writing code. It involved leading a team, collaborating with multiple departments, and constantly adapting to new challenges. Throughout this journey, I learned the importance of flexibility and communication—our modular design, cross-functional teamwork, and attention to detail ensured that no hardware change could disrupt the system’s performance.
Our approach—combining advanced tech, modularity, and strong collaboration—allowed us to create a control system that can adapt to any challenge. With 6DOF control, reliable networking, user-friendly interfaces, and the ability to seamlessly integrate new hardware, ROVIN is ready for the competition.